.NET Object Serialization
Have you ever wanted to send an object across application domains, save the object as a file or send it over the Internet to completely different service - then object serialization is for you. Serialization offers a way to describe object’s state(data) in a way that is understandable by other components. For example, object can be transformed into a XML or a Soap document, will get into output formats latter on. This is very useful if you are working on a big business application that contains huge objects with contains many references to other objects. By default class is not serializable and you need to explicitly state that you want to serialize it. In .NET it is very simple, just put [Serializable] attribute on the top of class.
[Serializable]
public class Student{ }
Serialization uses reflection to go over the object and serialize its fields, if it finds a reference to another objects, it check if the referenced object is serializable, if it is it walks over all the members and serializes them as well. It goes all the object’s hierarchy till everything is serialized. Sometimes same object can be references in multiple places, in that case it will not serialize the object again, but use previously serialized object. In case if an object is encounter that is not serializable the serialization exception will be thrown.
Example
[Serializable]
public class Student
{
public string Name;
public string Surname;
public int Age;
private string secret = "secretKey";
}
For example I will use the student class with three public fields and one private field. I left one field private to show you that some Serializers cannot access private fields.
Student student = new Student() { Name = "John", Age = 22 };
XmlSerializer serializer = new XmlSerializer(typeof(Student));
Stream stream = new MemoryStream();
serializer.Serialize(stream, student);
Code above is straight forwards, initialize a new student object with only two fields populated, one is left out to see how NULL values are serialized. The XMLSerializer requires one argument - the type what you are about to serialize. Output of a Serializer is sent to a stream, it could be any stream that is derived from the Stream class. In this case I used MemoryStream.
<?xml version="1.0"?>
<Student xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:xsd="http://www.w3.org/2001/XMLSchema">
<Name>John</Name>
<Age>22</Age>
</Student>
XML above is the content from the memory stream, as you can see NULL and private properties are left out. Desirialization is pretty straight forwards, just call Deserialize method and point it to the stream that hold serialized object. Make sure that stream position is set at the start of XML.
stream.Position = 0;
Student newStudent = (Student)serializer.Deserialize(stream);
Sometimes there will be properties which should be left out from serialization, those properties would hold temporary values. For example student sessionID for a student object. In that case it is very easy to mark a property not serializable.
[NonSerialized]
public int SessionID;
Types
XML is not the only way to serialize an object in C#. There are formatters that can serialize object to a binary or SOAP. The biggest different between formatters and XmlSerializer is that the formatter:
- Will serialize private properties
- Will not call the object’s constructor during deserialization process
- Single formatter can serialize different object types, it is not bound to a single object type.
BinaryFormatter binaryFormatter = new BinaryFormatter();
binaryFormatter.Serialize(stream, student);
stream.Position = 0;
Student newStudent = (Student)binaryFormatter.Deserialize(stream);
SOAP formatter is exactly the same, just add it as a reference to your project.
Performance
To see which performs better out of these three serializers, I extended Students class with a new Student property, so a student object can have a child object. By doing this it is possible to create a long chain of objects to see how each serializer performs. Graph below shows that formatters are faster then XML serializer. While formatters grown linearly, XML serializer grows exponentially.
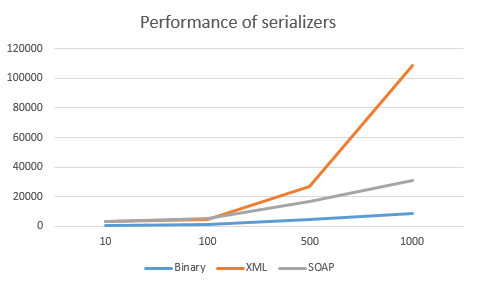